Validating URLs and email addresses in PHP
•
1 min read
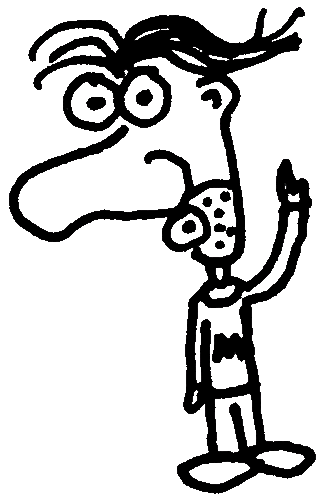
Heads up! This post was written in 2013, so it may contain information that is no longer accurate. I keep posts like this around for historical purposes and to prevent link rot, so please keep this in mind as you're reading.
— Cory
This is a simple method for validating both email addresses and URLs. Using PHP's filter_var()
function, it's actually very easy and doesn't require regular expressions. The following wrapper functions force a true boolean response, so you can use them safely in your logic.
Email addresses #
function is_email($email) {
return filter_var($email, FILTER_VALIDATE_EMAIL) !== false;
}
URLs #
function is_url($url) {
return filter_var($url, FILTER_VALIDATE_URL) !== false;
}
If you choose to not use the wrappers, just remember that filter_var()
doesn't always return a boolean value. It will return the original string on success, and false
on failure..