Swapping variables with JavaScript
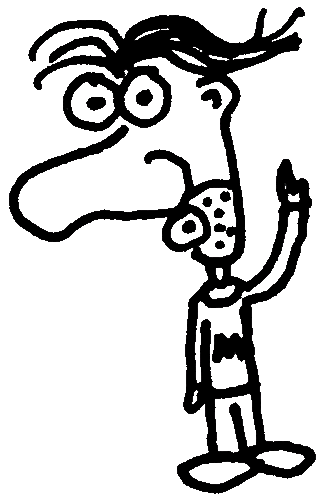
Heads up! This post was written in 2014, so it may contain information that is no longer accurate. I keep posts like this around for historical purposes and to prevent link rot, so please keep this in mind as you're reading.
— Cory
Swapping the value of two variables normally takes three lines and a temporary variable. What if I told you there was an easier way to do this with JavaScript?
Traditional method #
The goal is to swap the values of a
and b
. The textbook method for doing this looks something like this:
var a = 1;
var b = 2;
var c;
c = a;
a = b;
b = c;
Of course, we've introduced another variable called c
to temporarily store the original value of a
during the swap. But can we do it without c
?
One-line method #
This trick uses an array to perform the swap. Take a second to wrap your head around it:
b = [a, a = b][0];
There are a few things happening here, so if you're still having trouble understanding how or why this works, I'll explain:
- We're utilizing an array where the first index is the value of
a
and the second index is the value ofb
a
is set to the value ofb
when the array is createdb
is set to the first index of the array, which is a- MAGIC
While this trick definitely saves you a few lines of code and a temp variable, be cautious when using it. It's doesn't help that whole code clarity thing.
Want to learn another cool way to swap variables? If you're a math buff, you'll like the xor trick.