Passing data from PHP to JavaScript
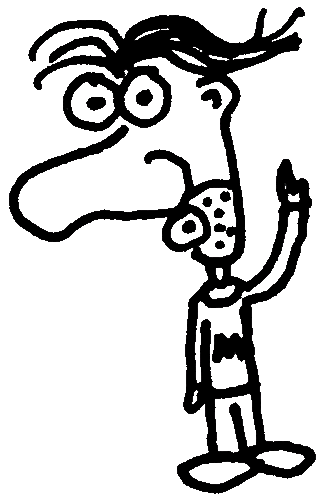
Heads up! This post was written in 2015, so it may contain information that is no longer accurate. I keep posts like this around for historical purposes and to prevent link rot, so please keep this in mind as you're reading.
— Cory
Have you ever needed to send a PHP variable, array, or object to JavaScript? It can get complicated trying to escape the output properly. Here's a way that always works—no escaping necessary.
Let's say we have the following variable in PHP:
$name = 'Bob Marley';
And we want to pass it to a JavaScript variable called name
. Here's the trick:
echo '<script>';
echo 'var name = ' . json_encode($name) . ';';
echo '</script>';
Using json_encode()
, you'll always get a properly formatted JavaScript object.
The same trick can be applied to other data types (e.g. integers, arrays, objects, etc.). The following passes an entire array from PHP to JavaScript:
$shirt = array(
'color' => 'blue',
'number' => 23,
'size' => 'XL'
);
echo '<script>';
echo 'var shirt = ' . json_encode($shirt) . ';';
echo '</script>';
The output looks like this:
<script>var shirt = {"color":"blue","number":23,"size":"XL"}</script>
Don't want it all on one line? Try this instead:
echo "<script>\n";
echo 'var shirt = ' . json_encode($shirt, JSON_PRETTY_PRINT) . ';';
echo "\n</script>";
The output is a bit easier to read:
<script>
var shirt = {
"color": "blue",
"number": 1000,
"size": "XL"
};
</script>
A simple trick, but nevertheless useful.