Parsing URLs in JavaScript
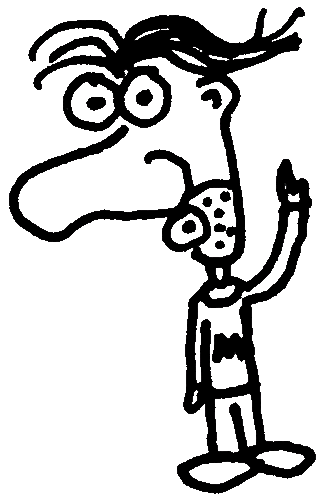
Heads up! This post was written in 2013, so it may contain information that is no longer accurate. I keep posts like this around for historical purposes and to prevent link rot, so please keep this in mind as you're reading.
— Cory
There's an excellent trick to parsing URLs in JavaScript, which was introduced last year by John Long over on GitHub. This technique works great, but the resulting search
property will be a raw query string. This isn't very useful if you need to access certain variables in said query string. Thus, the following function expands on this paradigm, providing an additional property that contains an object based on the original query string.
function parseURL(url) {
const parser = document.createElement('a');
const searchObject = {};
// Let the browser do the work
parser.href = url;
// Convert query string to object for convenience
const queries = parser.search.replace(/^\?/, '').split('&');
for (let i = 0; i < queries.length; i++) {
const split = queries[i].split('=');
searchObject[split[0]] = split[1];
}
return {
protocol: parser.protocol,
host: parser.host,
hostname: parser.hostname,
port: parser.port,
pathname: parser.pathname,
search: parser.search,
searchObject: searchObject,
hash: parser.hash
};
}
Let's try it!
const url = parseURL('https://example.com/index.html?q=test');
console.log(url);
And here's the result:
{
protocol: 'https:',
host: 'example.com',
hostname: 'example.com',
port: '',
pathname: '/index.html',
search: '?q=test',
searchObject: {
q: 'test'
},
hash: ''
}