Detecting mobile devices with JavaScript
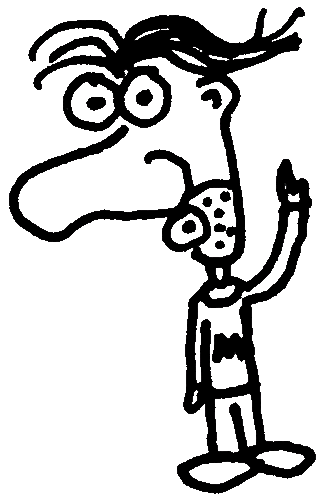
Heads up! This post was written in 2011, so it may contain information that is no longer accurate. I keep posts like this around for historical purposes and to prevent link rot, so please keep this in mind as you're reading.
— Cory
While I understand and value the concept of feature detection over browser detection, sometimes the need for knowing whether or not we're dealing with a mobile device arises. For in-depth device checking, you can rely on a complex library such as The MobileESP Project. But for simpler applications, the following snippet can be useful.
var isMobile = {
Android: function() {
return navigator.userAgent.match(/Android/i);
},
BlackBerry: function() {
return navigator.userAgent.match(/BlackBerry/i);
},
iOS: function() {
return navigator.userAgent.match(/iPhone|iPad|iPod/i);
},
Opera: function() {
return navigator.userAgent.match(/Opera Mini/i);
},
Windows: function() {
return navigator.userAgent.match(/IEMobile/i);
},
any: function() {
return (
isMobile.Android() ||
isMobile.BlackBerry() ||
isMobile.iOS() ||
isMobile.Opera() ||
isMobile.Windows()
);
}
};
While this doesn't account for all mobile platforms, it will pick up the most popular ones out there.
Examples #
To check to see if the user is on any of the supported mobile devices:
if (isMobile.any()) {
alert('Mobile');
}
To check to see if the user is on a specific mobile device:
if (isMobile.iOS()) {
alert('iOS');
}