A clean fade-in effect for webpages
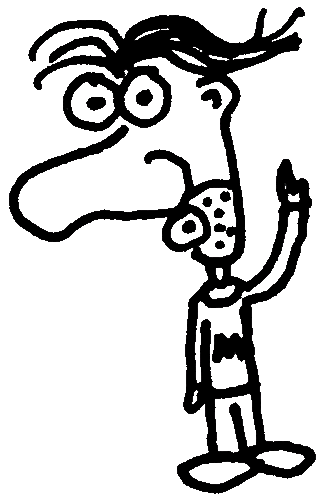
Heads up! This post was written in 2015, so it may contain information that is no longer accurate. I keep posts like this around for historical purposes and to prevent link rot, so please keep this in mind as you're reading.
— Cory
Here's a nice way to fade your pages in using CSS and a bit of JavaScript. The solution is clean and smooth, with no flickering on load. If JavaScript is disabled, the page will still load but the fade effect will not occur.
How it works #
This trick works by adding the fade-out
class to your body
with a script, then removing it when the page is done loading. The fade effect is handled completely with CSS. We're only using JavaScript to add/remove the class, which will ensure the page still displays if scripts are disabled.
The CSS #
We start with two very simple styles for the body:
body {
opacity: 1;
transition: 1s opacity;
}
body.fade-out {
opacity: 0;
transition: none;
}
The JavaScript #
Now we need to add the fade-out
class to the body as soon as the page loads. We can do this with an inline script right after the opening <body>
tag:
<script>
document.body.classList.add('fade-out');
</script>
The inline script is necessary (as opposed to a script loaded from a separate file) to prevent flickering, as the body would otherwise be briefly visible until the extra script is downloaded by the browser.
Now we just need to remove the fade-out
class from the body once the page is loaded.
window.addEventListener('DOMContentLoaded', () => {
document.body.classList.remove('fade-out');
});
Changing the fade color #
By default, the user will see a blank white screen until the page fades in. This is customizable through the html
selector:
html {
background-color: black;
}
Tips #
Don't get too carried away with this. It's a useful effect for loading something like a homepage, but it can quickly get annoying if you apply it to every page. Keep it subtle. Users shouldn't actually notice it—it should simply be a pleasant part of their experience.
This is a really helpful trick if you need to wait for an image or video to load. However, in that case you'll probably want to add a loader. To achieve this, simply add a wrapper element around everything except the loader and apply the fade-out
class to that instead of the body.