PHP functions to get and remove the file extension from a string
•
1 min read
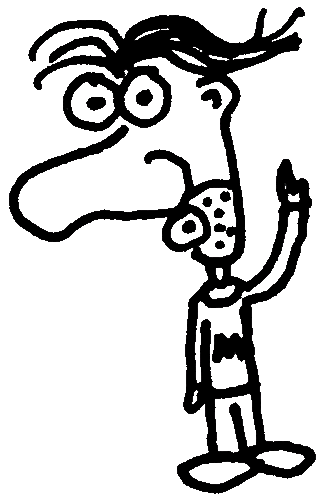
Heads up! This post was written in 2009, so it may contain information that is no longer accurate. I keep posts like this around for historical purposes and to prevent link rot, so please keep this in mind as you're reading.
— Cory
I use these regular expressions all the time, but it's much more convenient to have them both in convenient PHP functions.
// Returns only the file extension (without the dot)
function file_ext($filename) {
return preg_match('/\./', $filename) ? preg_replace('/^.*\./', '', $filename) : '';
}
// Returns the file name minus its extension
function file_ext_strip($filename){
return preg_replace('/.[^.]*$/', '', $filename);
}
You can also use the built-in pathinfo
function to achieve the same result:
$filename = '/path/to/file.ext';
echo pathinfo($filename)['extension']; // "ext"
echo pathinfo($filename)['filename']; // "file"