Select all, select none, and invert selection with jQuery
•
1 min read
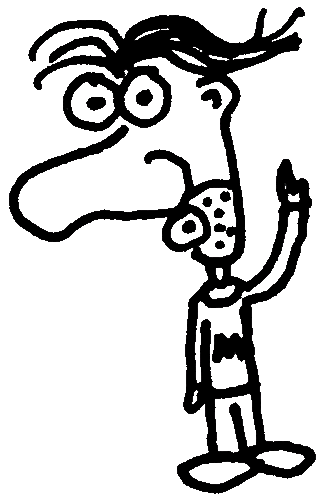
Heads up! This post was written in 2007, so it may contain information that is no longer accurate. I keep posts like this around for historical purposes and to prevent link rot, so please keep this in mind as you're reading.
— Cory
A demonstration of selecting checkboxes using jQuery.We start off with a bunch of checkboxes:
<fieldset id="group_1">
<input type="checkbox" name="numbers[]" value="0" />
<input type="checkbox" name="numbers[]" value="1" />
<input type="checkbox" name="numbers[]" value="2" />
<input type="checkbox" name="numbers[]" value="3" />
<input type="checkbox" name="numbers[]" value="4" />
<input type="checkbox" name="numbers[]" value="5" />
<input type="checkbox" name="numbers[]" value="6" />
<input type="checkbox" name="numbers[]" value="7" />
<input type="checkbox" name="numbers[]" value="8" />
<input type="checkbox" name="numbers[]" value="9" />
</fieldset>
Now, we add some links:
<a rel="group_1" href="#select_all">Select All</a>
<a rel="group_1" href="#select_none">Select None</a>
<a rel="group_1" href="#invert_selection">Invert Selection</a>
The rel
attribute is equal to the ID of the containing element of the checkbox group. In this example the containing element is a fieldset
, but it could be a DIV
, P
, UL
, etc.
Now, we add behaviors using jQuery:
<script type="text/javascript">
$(document).ready(function() {
// Select all
$("a[href='#select_all']").click(function() {
$("#" + $(this).attr('rel') + " input[type='checkbox']").attr('checked', true);
return false;
});
// Select none
$("a[href='#select_none']").click(function() {
$("#" + $(this).attr('rel') + " input[type='checkbox']").attr('checked', false);
return false;
});
// Invert selection
$("a[href='#invert_selection']").click(function() {
$("#" + $(this).attr('rel') + " input[type='checkbox']").each(function() {
$(this).attr('checked', !$(this).attr('checked'));
});
return false;
});
});
</script>
To add the same functionality to another group of checkboxes, create more links and adjust the rel
attribute accordingly:
<a rel="group_2" href="#select_all">Select All</a>
<a rel="group_2" href="#select_none">Select None</a>
<a rel="group_2" href="#invert_selection">Invert Selection</a>
<a rel="group_3" href="#select_all">Select All</a>
<a rel="group_3" href="#select_none">Select None</a>
<a rel="group_3" href="#invert_selection">Invert Selection</a>