A better way to write config files in PHP
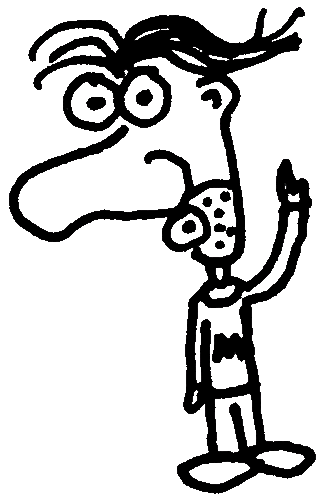
Heads up! This post was written in 2016, so it may contain information that is no longer accurate. I keep posts like this around for historical purposes and to prevent link rot, so please keep this in mind as you're reading.
— Cory
How many times have you seen something like this in a config file?
$db_host = 'localhost';
$db_name = 'somedb';
$db_user = 'someuser';
$db_pass = 'somepass';
Then, of course, it gets included and the variables are referenced as globals:
include('config.php');
echo $db_host; // 'localhost'
A better way #
I'm not a fan of using global variables when they can be avoided, so here's an alternative that gives you much more flexibility with your config files.
return [
'host' => 'localhost',
'name' => 'somedb',
'user' => 'someuser',
'pass' => 'somepass'
];
Simply returning the array allows you to include it into any variable you choose, which is much nicer than cluttering the global namespace with your config.
$database = include('config.php');
echo $database['host']; // 'localhost'
Of course, you can return any data you want with this approach — even a multidimensional array.
return [
'database' => [
'host' => 'localhost',
'name' => 'somedb',
'user' => 'someuser',
'pass' => 'somepass'
],
'other-stuff' => ...
];
This is by far my favorite way to write config files in PHP.